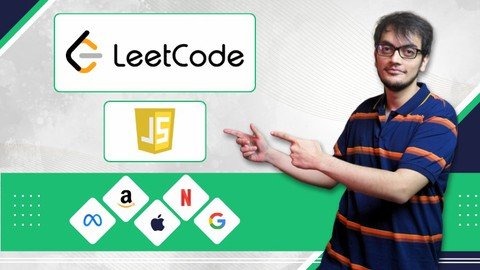
Published 7/2023
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 8.14 GB | Duration: 13h 57m
Mastering Leetcode In jаvascript - Step By Step
What you'll learn
Top 100 Leetcode Practice Problems
MAANG Interview Problems On Leetcode
Leetcode Practice Problems On Dynamic Programming, Greedy Algorithms in jаvascript
Leetcode Practice Problems On Graphs, Trees, Backtracking in jаvascript
Leetcode Practice Problems On Arrays, Sliding Window, Two Pointer, Ad hoc Problems in jаvascript
Requirements
Basic Coding Experience with topics like for loops, arrays
Description
The "Mastering the Top 100 Leetcode Problems" course is a comprehensive training program designed to help you excel in coding interviews by focusing on the top 100 Leetcode problems. Leetcode is a well-known platform that offers a vast collection of coding challenges frequently used by tech companies during their hiring process.In this course, we will tackle the most frequently encountered problems in coding interviews. Each problem will be thoroughly analyzed, providing you with valuable insights into the underlying concepts and problem-solving techniques. You will learn how to approach problems systematically, break them down into smaller manageable tasks, and devise efficient algorithms to solve them.A key aspect of this course is the live implementation of code. Each problem will be demonstrated in real-time, allowing you to witness the coding process firsthand. This practical approach will help solidify your understanding and improve your coding skills. You will gain insights into efficient coding practices, optimization techniques, and common pitfalls to avoid.We will go over each of the problems in extreme detail, going through the thought process, and live implementation for the code.To support your learning journey, the course will provide code sample files accompanying the video lectures.These resources will serve as valuable references and guides, assisting you in implementing the solutions effectively.
Overview
Section 1: Arrays and Techniques Based On Arrays
Lecture 1 Practice Problem 1 - Maximum Sum Subarray
Lecture 2 Practice Problem 2 - Best Time To Buy And Sell A Stock
Lecture 3 Practice Problem 3 - Majority Element
Lecture 4 Practice Problem 4 - Move Zeroes
Lecture 5 Practice Problem 5 - Two Sum II Input Array Is Sorted
Lecture 6 Practice Problem 6 - Trapping Rain Water
Lecture 7 Practice Problem 7 - Sort Colors
Lecture 8 Practice Problem 8 - Maximum Product Subarray
Lecture 9 Practice Problem 9 - Product Of Array Except Self
Lecture 10 Practice Problem 10 - Sliding Window Maximum
Lecture 11 Practice Problem 11 - Rotate Array
Lecture 12 Practice Problem 12 - Max Consecutive Ones
Lecture 13 Practice Problem 13 - Set Matrix Zeroes
Lecture 14 Practice Problem 14 - Container With Most Water
Lecture 15 Practice Problem 15 - Spiral Matrix
Lecture 16 Practice Problem 16 - Valid Sudoku
Section 2: Recursion & Backtracking
Lecture 17 Practice Problem 1 - Combination Sum
Lecture 18 Practice Problem 2 - Subsets
Lecture 19 Practice Problem 3 - N Queens
Section 3: Binary Search
Lecture 20 Practice Problem 1 - Search Insert Position
Lecture 21 Practice Problem 2 - Find First And Last Position Of Element In Sorted Array
Lecture 22 Practice Problem 3 - Search In A Rotated Sorted Array
Lecture 23 Practice Problem 4 - Koko Eating Bananas
Lecture 24 Practice Problem 5 - Magnetic Force Between Two Balls
Lecture 25 Practice Problem 6 - Find Minimum In A Rotated Sorted Array
Section 4: Binary Search Tree
Lecture 26 Practice Problem 1 - Kth Smallest Element In BST
Lecture 27 Practice Problem 2 - Validate Binary Search Tree
Lecture 28 Practice Problem 3 - Lowest Common Ancestor Of A Binary Search Tree
Section 5: Binary Trees
Lecture 29 Practice Problem 1 - Binary Tree InOrder Traversal
Lecture 30 Practice Problem 2 - Symmetric Tree
Lecture 31 Practice Problem 3 - Maximum Depth Of Binary Tree
Lecture 32 Practice Problem 4 - Invert Binary Tree
Lecture 33 Practice Problem 5 - Diameter Of A Binary Tree
Lecture 34 Practice Problem 6 - Binary Tree Level Order Traversal
Lecture 35 Practice Problem 7 - Path Sum
Lecture 36 Practice Problem 8 - Path Sum II
Lecture 37 Practice Problem 9 - Cousins In Binary Tree
Lecture 38 Practice Problem 10 - Maximum Level Sum Of Binary Tree
Lecture 39 Practice Problem 11 - Same Tree
Lecture 40 Practice Problem 12 - Construct Binary Tree From PreOrder And InOrder Traversal
Lecture 41 Practice Problem 13 - Subtree Of Another Tree
Lecture 42 Practice Problem 14 - Balanced Binary Tree
Lecture 43 Practice Problem 15 - Binary Tree Right Side View
Lecture 44 Practice Problem 16 - Count Good Nodes In Binary Tree
Lecture 45 Practice Problem 17 - Sum Of Left Leaves
Lecture 46 Practice Problem 18 - Construct Binary Tree From Inorder And Postorder Traversal
Section 6: Bit Manipulation
Lecture 47 Practice Problem 1 - Single Number
Lecture 48 Practice Problem 2 - Counting Bits
Lecture 49 Practice Problem 3 - Find The Duplicate Number
Lecture 50 Practice Problem 4 - Divide Two Integers
Lecture 51 Practice Problem 5 - Missing Number
Lecture 52 Practice Problem 6 - Number Of 1 Bits
Lecture 53 Practice Problem 7 - Sum Of Two Integers
Lecture 54 Practice Problem 8 - Reverse Integer
Section 7: Dynamic Programming
Lecture 55 Practice Problem 1 - Climbing Stairs
Lecture 56 Practice Problem 2 - Jump Game
Lecture 57 Practice Problem 3 - Coin Change
Lecture 58 Practice Problem 4 - Target Sum
Lecture 59 Practice Problem 5 - Longest Common Subsequence
Lecture 60 Practice Problem 6 - House Robber
Lecture 61 Practice Problem 7 - Longest Increasing Subsequence
Lecture 62 Practice Problem 8 - Partition Equal Subset Sum
Lecture 63 Practice Problem 9 0-1 Matrix
Lecture 64 Practice Problem 10 - Integer Replacement
Lecture 65 Practice Problem 11 - Edit Distance
Lecture 66 Practice Problem 12 - Decode Ways
Lecture 67 Practice Problem 13 - House Robber II
Lecture 68 Practice Problem 14 - Min Cost Climbing Stairs
Lecture 69 Practice Problem 15 - Longest Palindromic Substring
Section 8: Graphs
Lecture 70 Practice Problem 1 - Course Schedule
Lecture 71 Practice Problem 2 - Number Of Islands
Lecture 72 Practice Problem 3 - Find The Town Judge
Lecture 73 Practice Problem 4 - Surrounded Regions
Lecture 74 Practice Problem 5 - Number Of Enclaves
Lecture 75 Practice Problem 6 - Number Of Connected Components In Undirected Graph
Section 9: Hash Table
Lecture 76 Practice Problem 1 - Two Sum
Lecture 77 Practice Problem 2 - Three Sum
Lecture 78 Practice Problem 3 - Longest Consecutive Sequence
Lecture 79 Practice Problem 4 - Contains Duplicate
Lecture 80 Practice Problem 5 - Valid Anagram
Section 10: Heaps
Lecture 81 Practice Problem 1 - Kth Largest Element In An Array
Lecture 82 Practice Problem 2 - Find Median From Data Stream
Lecture 83 Practice Problem 3 - Kth Largest Element In A Stream
Section 11: Linked Lists
Lecture 84 Practice Problem 1 - Intersection Of Two Linked Lists
Lecture 85 Practice Problem 2 - Merge Two Sorted Lists
Lecture 86 Practice Problem 3 - Linked List Cycle
Lecture 87 Practice Problem 4 - Reverse Linked List
Lecture 88 Practice Problem 5 - Palindrome Linked List
Lecture 89 Practice Problem 6 - Linked List Cycle II
Lecture 90 Practice Problem 7 - Find Middle Of The Linked List
Lecture 91 Practice Problem 8 - Remove Nth Node From End Of List
Lecture 92 Practice Problem 9 - Add Two Numbers
Section 12: Stacks
Lecture 93 Practice Problem 1 - Valid Paranthesis
Lecture 94 Practice Problem 2 - Min Stack
Lecture 95 Practice Problem 3 - Merge Intervals
Lecture 96 Practice Problem 4 - Next Greater Element II
Lecture 97 Practice Problem 5 - Evaluate Reverse Polish Notation
Section 13: Strings
Lecture 98 Practice Problem 1 - Minimum Add To Make Parantheses Valid
Lecture 99 Practice Problem 2 - Group Anagrams
Lecture 100 Practice Problem 3 - Longest Substring Without Repeating Characters
This course is for anyone practicing on Leetcode for coding interviews
Screenshots
https://nitroflare.com/view/D0DE17857841EF4/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part01.rar
https://nitroflare.com/view/18E1A4196198689/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part02.rar
https://nitroflare.com/view/75223C3038E2516/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part03.rar
https://nitroflare.com/view/7A7C6DA085F2C57/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part04.rar
https://nitroflare.com/view/E71CBC94633925C/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part05.rar
https://nitroflare.com/view/D204F9487580DBE/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part06.rar
https://nitroflare.com/view/4873C8B70A573B9/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part07.rar
https://nitroflare.com/view/2BE0FBF8E03AA56/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part08.rar
https://nitroflare.com/view/EF95DD4B4D46937/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part09.rar
https://rapidgator.net/file/257309bce8d3c7a529f7c4d0288280d4/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part09.rar.html
https://rapidgator.net/file/c5466a8f62df25c7961d0a67f1d8bb4c/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part01.rar.html
https://rapidgator.net/file/7bd656941b3aef5ec4dc60e653585070/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part02.rar.html
https://rapidgator.net/file/6146782e90e26f83fcb699f8fb98c935/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part03.rar.html
https://rapidgator.net/file/305c3c5d3d45503b2a2ec6e45d4950b9/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part04.rar.html
https://rapidgator.net/file/f5906a8816a31549672fa7fea25d5c17/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part05.rar.html
https://rapidgator.net/file/cbe11a58d1382ea96558e04f40dadb74/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part06.rar.html
https://rapidgator.net/file/dd60c41e7ecd3fca20959863a84be70e/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part07.rar.html
https://rapidgator.net/file/a3e312b1b7aa33bfee0247a4c9a93f1d/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part08.rar.html
https://nitroflare.com/view/18E1A4196198689/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part02.rar
https://nitroflare.com/view/75223C3038E2516/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part03.rar
https://nitroflare.com/view/7A7C6DA085F2C57/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part04.rar
https://nitroflare.com/view/E71CBC94633925C/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part05.rar
https://nitroflare.com/view/D204F9487580DBE/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part06.rar
https://nitroflare.com/view/4873C8B70A573B9/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part07.rar
https://nitroflare.com/view/2BE0FBF8E03AA56/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part08.rar
https://nitroflare.com/view/EF95DD4B4D46937/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part09.rar
https://rapidgator.net/file/257309bce8d3c7a529f7c4d0288280d4/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part09.rar.html
https://rapidgator.net/file/c5466a8f62df25c7961d0a67f1d8bb4c/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part01.rar.html
https://rapidgator.net/file/7bd656941b3aef5ec4dc60e653585070/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part02.rar.html
https://rapidgator.net/file/6146782e90e26f83fcb699f8fb98c935/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part03.rar.html
https://rapidgator.net/file/305c3c5d3d45503b2a2ec6e45d4950b9/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part04.rar.html
https://rapidgator.net/file/f5906a8816a31549672fa7fea25d5c17/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part05.rar.html
https://rapidgator.net/file/cbe11a58d1382ea96558e04f40dadb74/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part06.rar.html
https://rapidgator.net/file/dd60c41e7ecd3fca20959863a84be70e/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part07.rar.html
https://rapidgator.net/file/a3e312b1b7aa33bfee0247a4c9a93f1d/Mastering_Leetcode_In_jаvascript_Top_100_Problems.part08.rar.html
