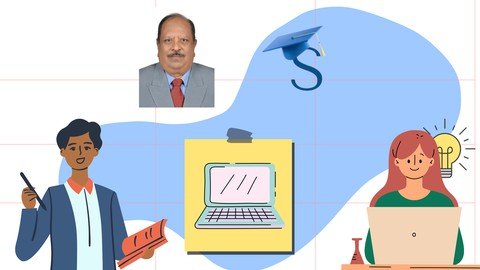
Published 10/2023
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 7.83 GB | Duration: 19h 24m
STL Containers, Iterators and Algorithms, Lambda Expressions, C++11/14/17/20 Features, Inheritance and Polymorphism
What you'll learn
Master C++ syntax, data types, and variables, including integer, floating-point, and character types.
Learn how to use if statements, switch statements, and loops (for, while, do-while) for control flow in programs.
Understand how to define and call functions, including function prototypes and parameter passing.
Get an introduction to OOP concepts such as classes, objects, encapsulation, and constructors.
Learn how to work with pointers and references, including dynamic memory allocation with new and delete.
Understand file input and output operations in C++ using file streams.
Explore the core components of the STL, such as vectors, lists, and algorithms, and how to use them effectively.
Dive deeper into OOP with topics like inheritance, polymorphism, and method overriding.
Understand template classes and functions to write generic code.
Learn about multithreading and how to write concurrent C++ programs using threads and synchronization primitives.
Gain an in-depth understanding of various STL containers (e.g., maps, sets) and advanced algorithms.
Explore advanced OOP topics like abstract classes, virtual functions, and multiple inheritance.
Master the use of lambda expressions for defining inline functions.
Stay up-to-date with the latest features and enhancements in modern C++ standards.
Apply your C++ knowledge to real-world projects, working on problem-solving and application development.
Requirements
No prior programming experience required.
Description
Are you ready to unlock the full potential of C++ programming? Welcome to the course entitled "Mastering C++ Online with Hands on Programming," an in-depth online course designed to take you on a journey from a beginner to an advanced C++ programmer.Course Overview:C plus plus is a powerful, versatile programming language used in various domains, from system-level programming to game development and beyond. Whether you're a complete novice or an experienced programmer looking to deepen your skills, this course is your gateway to mastering C plus plus.What You'll Learn: 1. C plus plus Fundamentals: Start with the basics. Learn about syntax, data types, variables, and control structures. Get comfortable writing your first programs.2. Object-Oriented Programming (OOP): Explore the world of OOP as you delve into classes, objects, inheritance, polymorphism, and encapsulation. Discover how to design elegant, maintainable code.3. Advanced Topics: Dive into advanced C plus plus topics such as templates, exceptions, and memory management. Learn to write efficient, error-resistant code.4. STL and Modern C plus plus: Uncover the power of the Standard Template Library (STL) and discover modern features like lambda expressions, smart pointers, and more.5. Concurrency and Multithreading: Master the art of multithreading and concurrent programming in C plus plus. 6. Best Practices and Optimization: Learn industry best practices and techniques for writing clean, efficient code. Profile and optimize your programs.7. Real-World Projects: Apply your knowledge by working on real-world projects. Develop practical skills that you can use immediately in your career or personal projects.Why Choose This Course:Comprehensive Curriculum: Our course covers everything from the fundamentals to advanced topics, ensuring you have a well-rounded understanding of the languagePractical Learning: Hands-on coding exercises, quizzes, and real-world projects give you practical experience to reinforce your learning.Experienced Instructor: Learn from experienced developer who is passionate about teaching and helping you succeed.Flexibility: Study at your own pace, with 24/7 access to course materials. Whether you're a full-time student or a working professional, this course fits your schedule.Career Opportunities: The language skills are in high demand across various industries. Gain the skills and confidence you need to pursue a rewarding career.Who Should Enroll:Beginners with no prior programming experienceNovice programmers looking to expand their skillsComputer science students and professionalsGame developers, system programmers, and embedded systems developersSoftware engineers seeking proficiency in the languageAnyone interested in mastering C plus plus for personal or career growthDon't miss this opportunity to become a C++ expert. Enroll now and embark on your journey to mastering C++ programming today!Note: Course content is regularly updated to keep up with the latest C++ standards and industry trends.
Overview
Section 1: Introduction
Lecture 1 Introduction
Lecture 2 Evolution of C++
Lecture 3 C++ Program Development, Program Development Steps, Code::Blocks, Sample Program
Lecture 4 Data Types
Lecture 5 Arrays
Lecture 6 Operators
Lecture 7 Branching and Iterations
Section 2: Introduction to C++ Standard Library and user defined types
Lecture 8 Reusable Software Development
Lecture 9 Strings and Vectors
Lecture 10 Functions
Lecture 11 Advanced Function Concepts
Lecture 12 Pointers
Lecture 13 Structures
Lecture 14 Structures & Vectors
Lecture 15 Dynamic Allocation of Memory
Section 3: Introduction to Object Oriented Programming
Lecture 16 Introduction to Classes
Lecture 17 Parameterized Constructor
Lecture 18 Memmber Functions
Lecture 19 friend function
Lecture 20 Constructors & Destructors
Lecture 21 Function & Operator Overloading – part 1
Lecture 22 Operator Overloading – part 2
Lecture 23 Inheritance Basics
Lecture 24 Multilevel Inheritance
Lecture 25 Types of Inheritance
Lecture 26 Reusing Code - Java, Python and C++
Lecture 27 Lesson 11 BBuilding Reusable Code with C++
Lecture 28 Lesson 11 C Pointers to Objects and Pointer Array in Reuse
Section 4: Advanced topics in Object Oriented Programming
Lecture 29 Virtual Functions and Polymorphism
Lecture 30 Abstract Class
Lecture 31 Container Class
Lecture 32 Function Templates
Lecture 33 Class Templates
Lecture 34 Advanced Concepts of Templates
Lecture 35 C++ Revisions
Lecture 36 Type Inference - auto
Lecture 37 Universal Reference- auto&&, decltype
Lecture 38 use of universal reference auto&&
Lecture 39 Lambdas
Section 5: Input/Output and Exception Handling
Lecture 40 Standard Input/Output
Lecture 41 Formatted Output
Lecture 42 File Write and Read
Lecture 43 File Mode Parameters
Lecture 44 String Stream
Lecture 45 CSV Files
Lecture 46 Exception handling intro'
Lecture 47 More Custom Exception classes
Lecture 48 Standard Exception Classes
Section 6: Standard Template Library
Lecture 49 C++ Standard Library
Lecture 50 Standard Template Library
Lecture 51 Vectors
Lecture 52 More on Vectors
Lecture 53 Lists
Lecture 54 Lists – Iterators and Algorithms
Lecture 55 Strings
Lecture 56 Deque
Lecture 57 Map
Lecture 58 Other Associative Containers
Lecture 59 Latest Additions to C++
Lecture 60 Function Objects & Bitsets
Lecture 61 Multithreading
Beginners with no programming experience,Individuals with some programming experience in other languages who want to learn C++ to expand their skillset or pursue specific projects.,Students enrolled in computer science programs who need to learn C++ as part of their coursework.,Programmers who are proficient in languages like Python, Java, or Ruby and want to learn C++ for its performance advantages or because they're entering a field where C++ is commonly used (e.g., game development, system programming).,Game developers who want to use C++ for game engine development, game programming, or optimization,Developers working on embedded systems and IoT applications where C++ is a common choice due to its control and efficiency.,System programmers: Those interested in low-level programming, operating system development, or working with hardware interfaces often need to learn C++.,Individuals aiming for roles where they need to design complex software systems and want a deep understanding of C++ to make informed architectural decisions.,Hobbyists and enthusiasts who have a passion for programming and want to explore C++ as a learning experience or for personal projects.,Job seekers who anticipate technical interviews that require C++ knowledge, especially for positions in tech companies or industries where C++ is prevalent.
Screenshots
https://rapidgator.net/file/dff35fdf2f485dfea449a654ec0082c7/Mastering_Latest_C_Online_With_Hands_On_Programming.part09.rar.html
https://rapidgator.net/file/6da251f0a5e374a67d96c753ede3de9f/Mastering_Latest_C_Online_With_Hands_On_Programming.part08.rar.html
https://rapidgator.net/file/f08887b22b49e4d819f54cae60e3b098/Mastering_Latest_C_Online_With_Hands_On_Programming.part07.rar.html
https://rapidgator.net/file/73bae5a2938c9b7a9a179358f1e2e81e/Mastering_Latest_C_Online_With_Hands_On_Programming.part06.rar.html
https://rapidgator.net/file/c0a54c79e3191e982886bffe4f9ce2e5/Mastering_Latest_C_Online_With_Hands_On_Programming.part05.rar.html
https://rapidgator.net/file/86b433e2528c624fdf35d6da32767008/Mastering_Latest_C_Online_With_Hands_On_Programming.part04.rar.html
https://rapidgator.net/file/f2f45839c81757b86ab751d298ab851d/Mastering_Latest_C_Online_With_Hands_On_Programming.part03.rar.html
https://rapidgator.net/file/968013e5739799eb1a6607ea4ed2c339/Mastering_Latest_C_Online_With_Hands_On_Programming.part02.rar.html
https://rapidgator.net/file/a3ec24ad698ad475dea7fe1ec9835565/Mastering_Latest_C_Online_With_Hands_On_Programming.part01.rar.html
https://nitroflare.com/view/152D90EEBA47C78/Mastering_Latest_C_Online_With_Hands_On_Programming.part09.rar
https://nitroflare.com/view/3BDBA7130E1240F/Mastering_Latest_C_Online_With_Hands_On_Programming.part08.rar
https://nitroflare.com/view/FA98B0ABC9DF421/Mastering_Latest_C_Online_With_Hands_On_Programming.part07.rar
https://nitroflare.com/view/15474D937344624/Mastering_Latest_C_Online_With_Hands_On_Programming.part06.rar
https://nitroflare.com/view/433B564D9DC390D/Mastering_Latest_C_Online_With_Hands_On_Programming.part05.rar
https://nitroflare.com/view/3F0638A47664371/Mastering_Latest_C_Online_With_Hands_On_Programming.part04.rar
https://nitroflare.com/view/22F4D1E6D4BBB8E/Mastering_Latest_C_Online_With_Hands_On_Programming.part03.rar
https://nitroflare.com/view/6349903E9BF705A/Mastering_Latest_C_Online_With_Hands_On_Programming.part02.rar
https://nitroflare.com/view/67F16D25F3C4C7F/Mastering_Latest_C_Online_With_Hands_On_Programming.part01.rar
https://rapidgator.net/file/6da251f0a5e374a67d96c753ede3de9f/Mastering_Latest_C_Online_With_Hands_On_Programming.part08.rar.html
https://rapidgator.net/file/f08887b22b49e4d819f54cae60e3b098/Mastering_Latest_C_Online_With_Hands_On_Programming.part07.rar.html
https://rapidgator.net/file/73bae5a2938c9b7a9a179358f1e2e81e/Mastering_Latest_C_Online_With_Hands_On_Programming.part06.rar.html
https://rapidgator.net/file/c0a54c79e3191e982886bffe4f9ce2e5/Mastering_Latest_C_Online_With_Hands_On_Programming.part05.rar.html
https://rapidgator.net/file/86b433e2528c624fdf35d6da32767008/Mastering_Latest_C_Online_With_Hands_On_Programming.part04.rar.html
https://rapidgator.net/file/f2f45839c81757b86ab751d298ab851d/Mastering_Latest_C_Online_With_Hands_On_Programming.part03.rar.html
https://rapidgator.net/file/968013e5739799eb1a6607ea4ed2c339/Mastering_Latest_C_Online_With_Hands_On_Programming.part02.rar.html
https://rapidgator.net/file/a3ec24ad698ad475dea7fe1ec9835565/Mastering_Latest_C_Online_With_Hands_On_Programming.part01.rar.html
https://nitroflare.com/view/152D90EEBA47C78/Mastering_Latest_C_Online_With_Hands_On_Programming.part09.rar
https://nitroflare.com/view/3BDBA7130E1240F/Mastering_Latest_C_Online_With_Hands_On_Programming.part08.rar
https://nitroflare.com/view/FA98B0ABC9DF421/Mastering_Latest_C_Online_With_Hands_On_Programming.part07.rar
https://nitroflare.com/view/15474D937344624/Mastering_Latest_C_Online_With_Hands_On_Programming.part06.rar
https://nitroflare.com/view/433B564D9DC390D/Mastering_Latest_C_Online_With_Hands_On_Programming.part05.rar
https://nitroflare.com/view/3F0638A47664371/Mastering_Latest_C_Online_With_Hands_On_Programming.part04.rar
https://nitroflare.com/view/22F4D1E6D4BBB8E/Mastering_Latest_C_Online_With_Hands_On_Programming.part03.rar
https://nitroflare.com/view/6349903E9BF705A/Mastering_Latest_C_Online_With_Hands_On_Programming.part02.rar
https://nitroflare.com/view/67F16D25F3C4C7F/Mastering_Latest_C_Online_With_Hands_On_Programming.part01.rar
